Introduction
In this article, we will learn how to use sessions in our .NET Core Web applications. I assume that your web application is already created.
Prerequisite
- .NET Core SDK
- Visual Studio
To use the session first we need to install Nuget namely “Microsoft.AspNetCore.Session”.
Go to Tools > NuGet Package Manager > Manage NuGet Packages For Solutions.
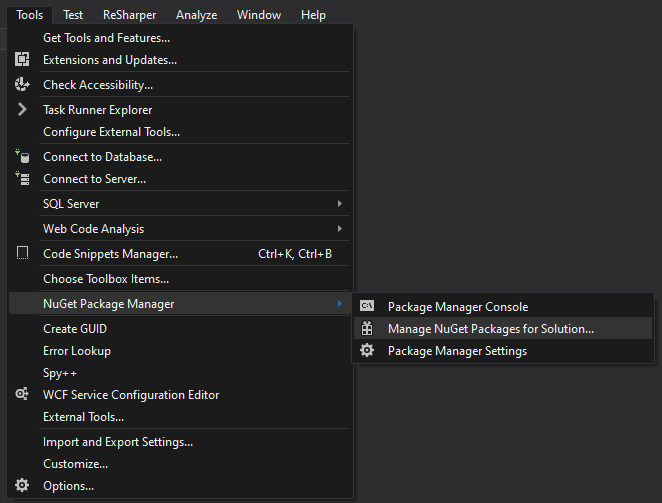
Click on Browse Tab and search for “Microsoft.AspNetCore.Session”. Click on search NuGet and click to Install button.

Now, We can use the session by following the below codes.
First we need to add a session in the ConfigureServices method of StartUp class.
- services.AddSession();
Now add a session in Configure method to configure the HTTP request pipeline.
- app.UseSession();
Startup class look like this
- public class Startup
- {
- public Startup(IConfiguration configuration)
- {
- Configuration = configuration;
- }
- public IConfiguration Configuration { get; }
- // This method gets called by the runtime. Use this method to add services to the container.
- public void ConfigureServices(IServiceCollection services)
- {
- services.Configure<CookiePolicyOptions>(options =>
- {
- // This lambda determines whether user consent for non-essential cookies is needed for a given request.
- options.CheckConsentNeeded = context => false;
- options.MinimumSameSitePolicy = SameSiteMode.None;
- });
- services.AddMvc().SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
- services.AddSession();
- services.AddHttpContextAccessor();
- }
- // This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
- public void Configure(IApplicationBuilder app, IHostingEnvironment env)
- {
- if (env.IsDevelopment())
- {
- app.UseDeveloperExceptionPage();
- }
- else
- {
- app.UseExceptionHandler("/Home/Error");
- app.UseHsts();
- }
- app.UseHttpsRedirection();
- app.UseStaticFiles();
- app.UseCookiePolicy();
- app.UseSession();
- app.UseMvc(routes =>
- {
- routes.MapRoute(
- name: "default",
- template: "{controller=Home}/{action=Index}/{id?}");
- });
- }
- }
To Set Session
- public IActionResult Index()
- {
- HttpContext.Session.SetString(SessionName, "Faisal Pathan");
- HttpContext.Session.SetInt32(SessionAge, 24);
- return View();
- }
To Get Session
- public IActionResult About()
- {
- Var userName = HttpContext.Session.GetString("UserName");
- var code = HttpContext.Session.GetInt32("Code");
- return View();
- }
We can also set the whole class/object in the session
To Set/Get Object as JSON from Session
- public IActionResult Index()
- {
- // some object
- object value = new object();
- // set object value in session
- HttpContext.Session.SetString("Key Name", JsonConvert.SerializeObject(value));
- // get object value in session
- var sesionValue = HttpContext.Session.GetString("Key Name);
- var sessionObj = value == null ? default(T) : JsonConvert.DeserializeObject<T>(sesionValue);
- return View();
- }
We also can create an Extention method to use sessions globally in our application.
- public static class SessionExtensions
- {
- public static void SetObjectAsJson(this ISession session, string key, object value)
- {
- session.SetString(key, JsonConvert.SerializeObject(value));
- }
- public static T GetObjectFromJson<T>(this ISession session, string key)
- {
- var value = session.GetString(key);
- return value == null ? default(T) : JsonConvert.DeserializeObject<T>(value);
- }
No hay comentarios:
Publicar un comentario